Today we will learn how to use the ESP32-S2 internal temperature sensor, using the Franzininho Wi-Fi board. Researching for this blog post was a bit intense, I was not able to make/find any example work at first.
Note: this code only works on some versions of the ESP32, check this blog post to see if your board qualifies.
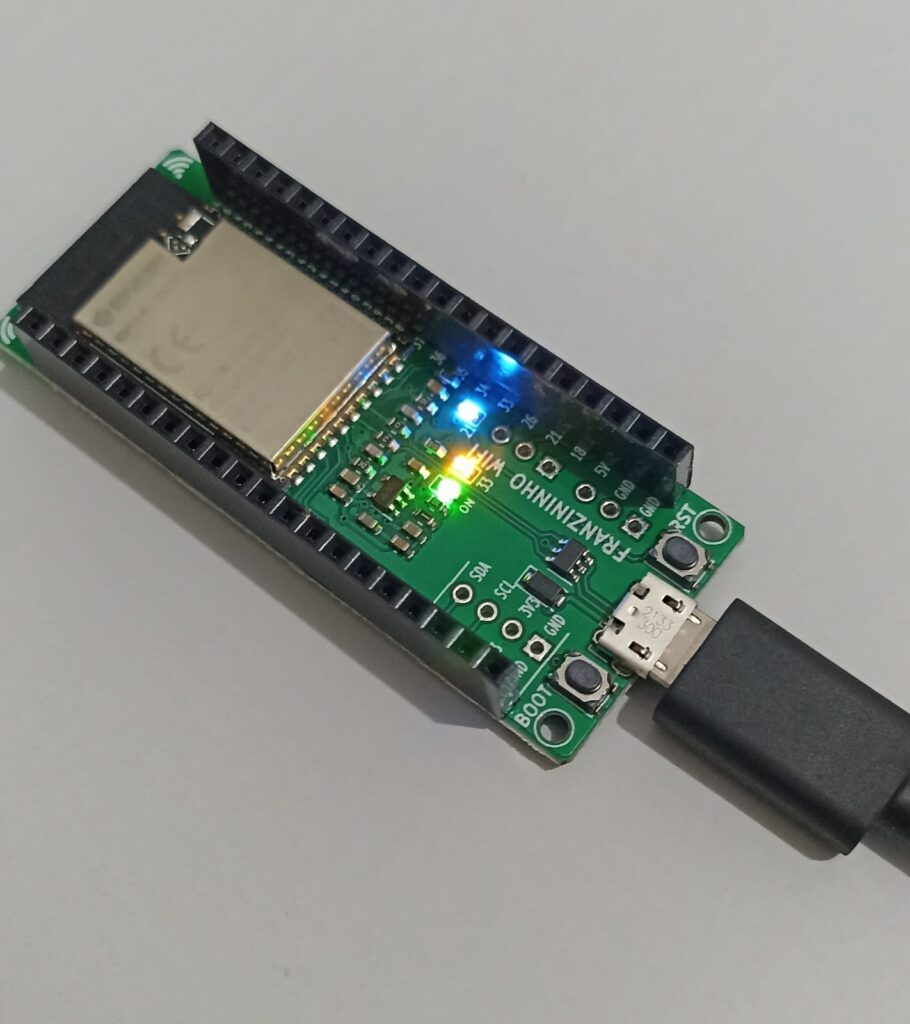
Finally this post carried part of the answer, a code simple enough to try. What I was finding interesting was that the
driver/temp_sensor.h
file was not present at the blog post, and the link to a GitHub leading to the file that was not the correct one. The complete code for the example (Arduino IDE) is seen below.
#include "C:\Users\Clovisf\Documents\Fritenlab\ESP32-S2-Franzinho-WiFi\temp_sensor.h"
void initTempSensor(){
temp_sensor_config_t temp_sensor = TSENS_CONFIG_DEFAULT();
temp_sensor.dac_offset = TSENS_DAC_L2; // TSENS_DAC_L2 is default; L4(-40°C ~ 20°C), L2(-10°C ~ 80°C), L1(20°C ~ 100°C), L0(50°C ~ 125°C)
temp_sensor_set_config(temp_sensor);
temp_sensor_start();
}
void setup() {
Serial.begin(115200);
initTempSensor();
}
void loop() {
Serial.print("Temperature: ");
float result = 0;
temp_sensor_read_celsius(&result);
Serial.print(result);
Serial.println(" °C");
delay(5000);
}
The actual “temp_sensor.h” file was found in this place. I had to download the content of the link (Whice is below), paste it into a notepad and name it “temp_sensor.h”. I then pointed the code the complete path to the file in my machine.
C:\Users\Clovisf\Documents\Fritenlab\ESP32-S2-Franzinho-WiFi\temp_sensor.h
Temp_sensor.h content:
/*
* SPDX-FileCopyrightText: 2010-2021 Espressif Systems (Shanghai) CO LTD
*
* SPDX-License-Identifier: Apache-2.0
*/
#pragma once
#include <stdint.h>
#include "esp_err.h"
#ifdef __cplusplus
extern "C" {
#endif
/**
* @brief temperature sensor range option.
*/
typedef enum {
TSENS_DAC_L0 = 0, /*!< offset = -2, measure range: 50℃ ~ 125℃, error < 3℃. */
TSENS_DAC_L1, /*!< offset = -1, measure range: 20℃ ~ 100℃, error < 2℃. */
TSENS_DAC_L2, /*!< offset = 0, measure range:-10℃ ~ 80℃, error < 1℃. */
TSENS_DAC_L3, /*!< offset = 1, measure range:-30℃ ~ 50℃, error < 2℃. */
TSENS_DAC_L4, /*!< offset = 2, measure range:-40℃ ~ 20℃, error < 3℃. */
TSENS_DAC_MAX,
TSENS_DAC_DEFAULT = TSENS_DAC_L2,
} temp_sensor_dac_offset_t;
/**
* @brief Configuration for temperature sensor reading
*/
typedef struct {
temp_sensor_dac_offset_t dac_offset; /*!< The temperature measurement range is configured with a built-in temperature offset DAC. */
uint8_t clk_div; /*!< Default: 6 */
} temp_sensor_config_t;
/**
* @brief temperature sensor default setting.
*/
#define TSENS_CONFIG_DEFAULT() {.dac_offset = TSENS_DAC_L2, \
.clk_div = 6}
/**
* @brief Set parameter of temperature sensor.
* @param tsens
* @return
* - ESP_OK Success
*/
esp_err_t temp_sensor_set_config(temp_sensor_config_t tsens);
/**
* @brief Get parameter of temperature sensor.
* @param tsens
* @return
* - ESP_OK Success
*/
esp_err_t temp_sensor_get_config(temp_sensor_config_t *tsens);
/**
* @brief Start temperature sensor measure.
* @return
* - ESP_OK Success
* - ESP_ERR_INVALID_ARG
*/
esp_err_t temp_sensor_start(void);
/**
* @brief Stop temperature sensor measure.
* @return
* - ESP_OK Success
*/
esp_err_t temp_sensor_stop(void);
/**
* @brief Read temperature sensor raw data.
* @param tsens_out Pointer to raw data, Range: 0 ~ 255
* @return
* - ESP_OK Success
* - ESP_ERR_INVALID_ARG `tsens_out` is NULL
* - ESP_ERR_INVALID_STATE temperature sensor dont start
*/
esp_err_t temp_sensor_read_raw(uint32_t *tsens_out);
/**
* @brief Read temperature sensor data that is converted to degrees Celsius.
* @note Should not be called from interrupt.
* @param celsius The measure output value.
* @return
* - ESP_OK Success
* - ESP_ERR_INVALID_ARG ARG is NULL.
* - ESP_ERR_INVALID_STATE The ambient temperature is out of range.
*/
esp_err_t temp_sensor_read_celsius(float *celsius);
#ifdef __cplusplus
}
#endif
Compiling and flashing the code to your ESP32-S2 Franzininho Wi-Fi, you can then look at the Arduino IDE console (serial monitor) and it will look like this.
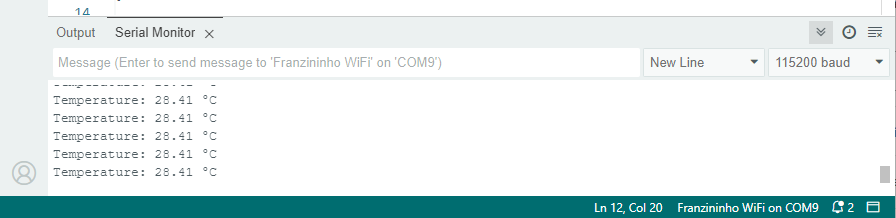
Temperature readings will be updated every five seconds. I noticed the readings are stable. That is a good sign when it comes to the reliability of the circuit and code.
That’s all for today, I hope you enjoyed it and it solved your problem.
Leave a Reply