Hello everyone, today we will make an example of ESP32-S2 publishing in the cloud to the Thingspeak service. We will heavily follow this tutorial to the Thingspeak part.
The temperature readings part will follow a tutorial I published here a while ago. What you have to do first is open the Arduino IDE and install the Thingspeak library.
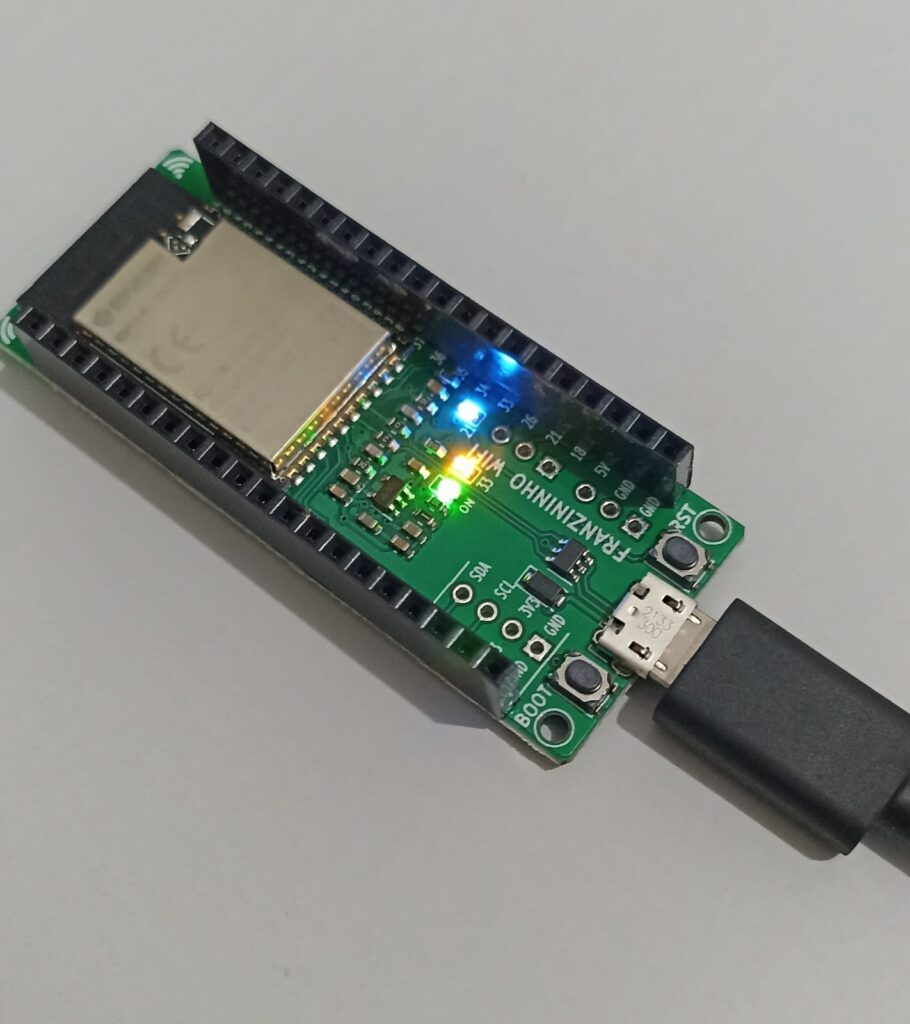
Go to “Tools > Manage Libraries..” and type “Thingspeak”. The Mathworks one is the correct. Now go to the Thingspeak website and create an account and a channel.
Thingspeak is an “iot analytics platform”, that in other words can receive and process data from remote devices.
Have your recently generated API key (on the Thingspeak website) in hand for the next step. Now see the Arduino code I made for the example.
It will read the ESP32-S2 internal temperature and send it to Thingspeak every 30 seconds. Note that you have to use your own “yourssid” and “yourpassword” for the Wi-Fi credentials.
Also the “myWriteAPIKey” is the write API key I comment above, “myChannelNumber” is the channel ID. All of this is available in the Thingspeak channel you just created.
"C:\Users\Clovisf\Documents\Fritenlab\ESP32-S2-Franzinho-WiFi\temp_sensor.h"
The above path (present in the code below) is to a file present in this post that makes the temperature readings work. Create such file with the code in the post above and point your own path.
/*
Adapted in 04/2024 by Clovis Fritzen for FritzenLab.net
Adapted from WriteSingleField Example from ThingSpeak Library (Mathworks)
Rui Santos
Complete project details at https://RandomNerdTutorials.com/esp32-thingspeak-publish-arduino/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*/
#include <WiFi.h>
#include "ThingSpeak.h"
#include "C:\Users\Clovisf\Documents\Fritenlab\ESP32-S2-Franzinho-WiFi\temp_sensor.h"
const char* ssid = "yourssid"; // your network SSID (name)
const char* password = "yourpassword"; // your network password
WiFiClient client;
unsigned long myChannelNumber = yourchannelID;
const char * myWriteAPIKey = "yourAPIwritekey";
// Timer variables
unsigned long lastTime = 0;
unsigned long timerDelay = 30000;
void initTempSensor(){
temp_sensor_config_t temp_sensor = TSENS_CONFIG_DEFAULT();
temp_sensor.dac_offset = TSENS_DAC_L2; // TSENS_DAC_L2 is default; L4(-40°C ~ 20°C), L2(-10°C ~ 80°C), L1(20°C ~ 100°C), L0(50°C ~ 125°C)
temp_sensor_set_config(temp_sensor);
temp_sensor_start();
}
void setup() {
Serial.begin(115200); //Initialize serial
initTempSensor();
WiFi.mode(WIFI_STA);
ThingSpeak.begin(client); // Initialize ThingSpeak
}
void loop() {
if ((millis() - lastTime) > timerDelay) {
// Connect or reconnect to WiFi
if(WiFi.status() != WL_CONNECTED){
Serial.print("Attempting to connect");
while(WiFi.status() != WL_CONNECTED){
WiFi.begin(ssid, password);
delay(5000);
}
Serial.println("\nConnected.");
}
float result = 0;
temp_sensor_read_celsius(&result);
// Write to ThingSpeak. There are up to 8 fields in a channel, allowing you to store up to 8 different
// pieces of information in a channel. Here, we write to field 1.
int x = ThingSpeak.writeField(myChannelNumber, 1, result, myWriteAPIKey);
if(x == 200){
Serial.println("Channel update successful.");
}
else{
Serial.println("Problem updating channel. HTTP error code " + String(x));
}
lastTime = millis();
}
}
I was able to collect the readings in the image below. Notice there is a gap between some points, it may be due to lost Wi-Fi connection. Also the temperature seems to be going up.
I suspect that is because the chip is heating due to Wi-Fi activity.
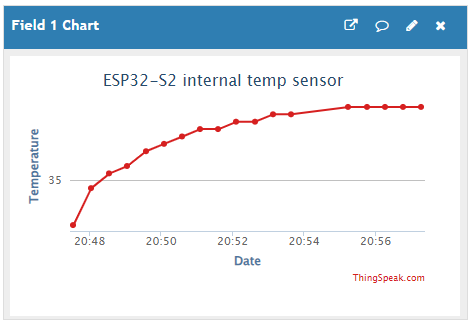
This is almost 13 hours of temperature data overnight, from 9PM to 10AM.
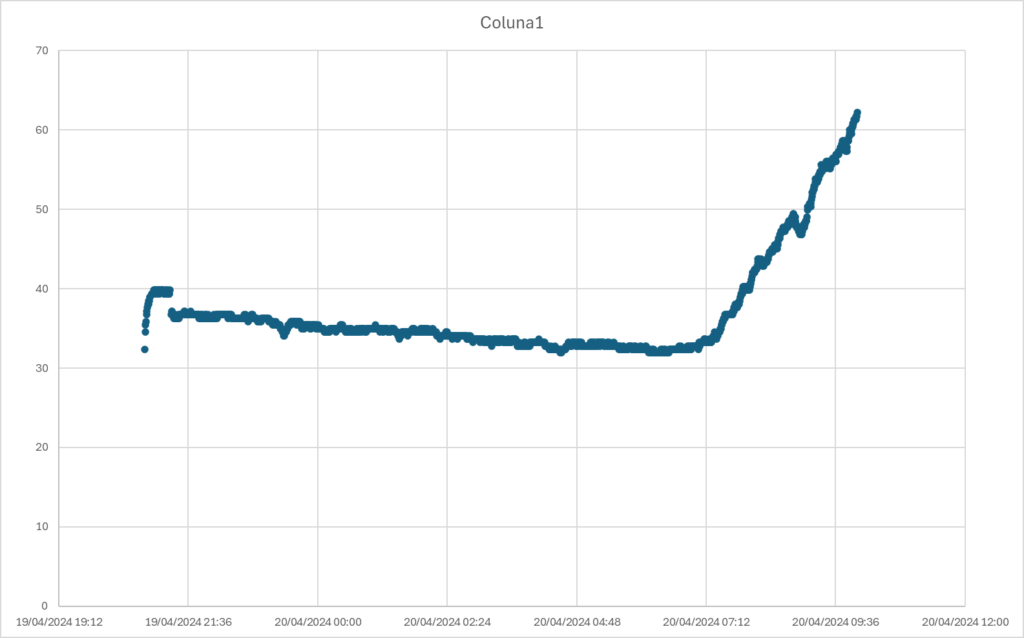
The code presented does not use delay(), so it does not block what it is doing. Instead it uses a technique that I explained here. I hope you enjoyed this article and look forward to your comment below.
Leave a Reply