Today we are going to implement an interesting function: blinkWithoutDelay with Raspberry Pi Pico and microPython. This function eliminates the need to use time.sleep() which leaves the program stuck waiting.
The idea is to use the time.ticks_us() function which provides the current time in microseconds. We keep counting until enough time passes between the current time (currentTime) and the previously captured time (oldTime).
In the code below, the value 1000000 means 1 million microseconds, or 1 second. I do a led.toggle() (turn it off and turn it on) on an external LED and on the onboard LED every second. The rest of the time the program is free to do whatever else it needs.
from machine import Pin
import time
led = machine.Pin(18, machine.Pin.OUT)
onboard = machine.Pin(25, machine.Pin.OUT)
oldTime = 0
currentTime = 0
led.off()
onboard.off()
while True:
currentTime = time.ticks_us()
if(currentTime - oldTime > 1000000):
oldTime = currentTime
led.toggle()
onboard.toggle()
Below is a gif of the code in operation. See that the external LED has a series 1k Ohm resistor.
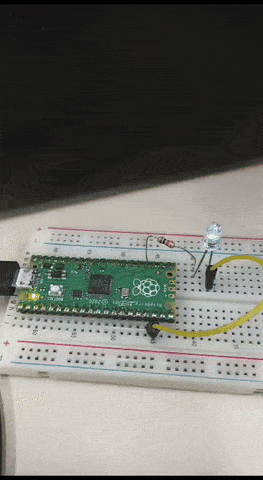
Now a code example that alternates between the LEDs.
from machine import Pin
import time
led = machine.Pin(18, machine.Pin.OUT)
onboard = machine.Pin(25, machine.Pin.OUT)
oldTime = 0
currentTime = 0
led.off()
onboard.off()
while True:
currentTime = time.ticks_us()
if(currentTime - oldTime > 1000000):
oldTime = currentTime
if(led.value() == True):
onboard.on()
led.off()
else:
onboard.off()
led.on()
Want to put more tasks in the same program? see the code below, where I put each of the two LEDs in a different task, in this case a different time.
One LED flashes every 1 second and the other every 250 milliseconds.
from machine import Pin
import time
led = machine.Pin(18, machine.Pin.OUT)
onboard = machine.Pin(25, machine.Pin.OUT)
oldTime = 0
oldTime2 = 0
currentTime = 0
led.off()
onboard.off()
while True:
currentTime = time.ticks_us()
if(currentTime - oldTime > 1000000):
oldTime = currentTime
onboard.toggle()
if(currentTime - oldTime2 > 250000):
oldTime2 = currentTime
led.toggle()
We are effectively executing more than one task (blinking two LEDs at different times) without taking up processor time with time.sleep(). There’s a little more about IO manipulation here.
Didn’t get the basics of microPython with Raspberry Pi Pico? read this article.
Leave a Reply