Today we are going to learn how to use the ultrasonic distance sensor HC-SR04 with ESP32, more specifically a dev board based on the Xiao ESP32-C6 I made myself. But it will work with any ESP32, really.
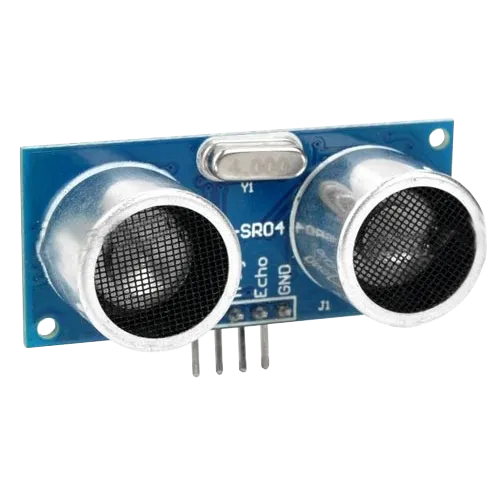
A ultrasonic distance sensor works by emitting a wave (not audible) and calculating the time it takes for the wave to reflect and come back to the sensor. The HC-SR04 specifically can measure between 2cm and 400cm. Its technical data is below:
Power Supply | 5V DC |
Working Current | 15 mA |
Working Frequency | 40 kHz |
Maximum Range | 4 meters |
Minimum Range | 2 cm |
Measuring Angle | 15ยบ |
Resolution | 0.3 cm |
Trigger Input Signal | 10uS TTL pulse |
Echo Output Signal | TTL pulse proportional to the distance range |
Dimensions | 45mm x 20mm x 15mm |
It is a very well known sensor can (and is) be used to various applications. I have seen some automated litter boxes that open automatically when you approximate your hand of it. There are also robots that navigate themselves inside a house, you name it.
Code for Arduino software
Code comes from here, a blog I like a lot. It defines the sound speed then calculates distance based on time, which comes from the pulseIn() function.
/*********
Rui Santos
Complete project details at https://RandomNerdTutorials.com/esp32-hc-sr04-ultrasonic-arduino/
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files.
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
*********/
const int trigPin = D5;
const int echoPin = D6;
//define sound speed in cm/uS
#define SOUND_SPEED 0.034
#define CM_TO_INCH 0.393701
long duration;
float distanceCm;
float distanceInch;
void setup() {
Serial.begin(115200); // Starts the serial communication
pinMode(trigPin, OUTPUT); // Sets the trigPin as an Output
pinMode(echoPin, INPUT); // Sets the echoPin as an Input
}
void loop() {
// Clears the trigPin
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Sets the trigPin on HIGH state for 10 micro seconds
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Reads the echoPin, returns the sound wave travel time in microseconds
duration = pulseIn(echoPin, HIGH);
// Calculate the distance
distanceCm = duration * SOUND_SPEED/2;
// Convert to inches
distanceInch = distanceCm * CM_TO_INCH;
// Prints the distance in the Serial Monitor
Serial.print("Distance (cm): ");
Serial.println(distanceCm);
Serial.print("Distance (inch): ");
Serial.println(distanceInch);
delay(1000);
}
Schematic diagram is as follow. Notice the only two pins/inputs: trigger connected to pin D5 and Echo connected to pin D6. In my experience it works well being supplied with 3.3V, but you only find it being supplied with 5V on the internet. Then I recommend you supply it with 5V, to be safe.
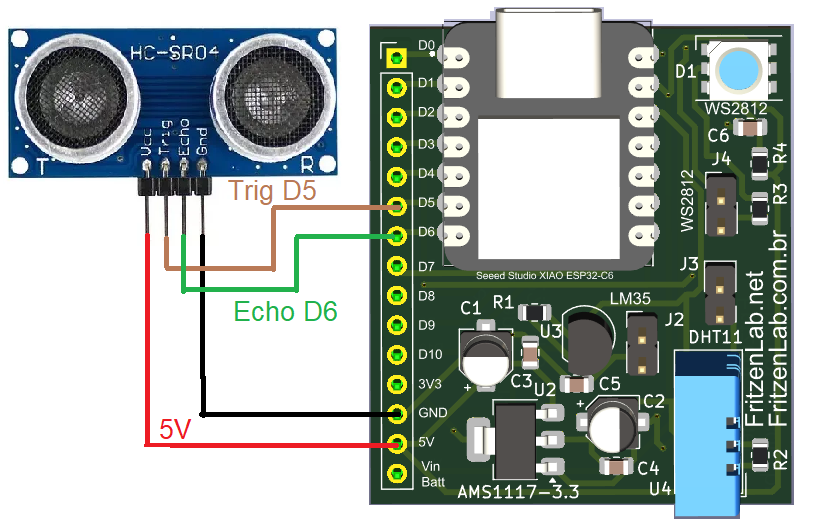
Results/testing
I have placed a 20cm ruler in front of the ultrasonic sensor, as a way to measure and compare distances. All distance information is sent to the Arduino IDE serial monitor via USB/serial interface.
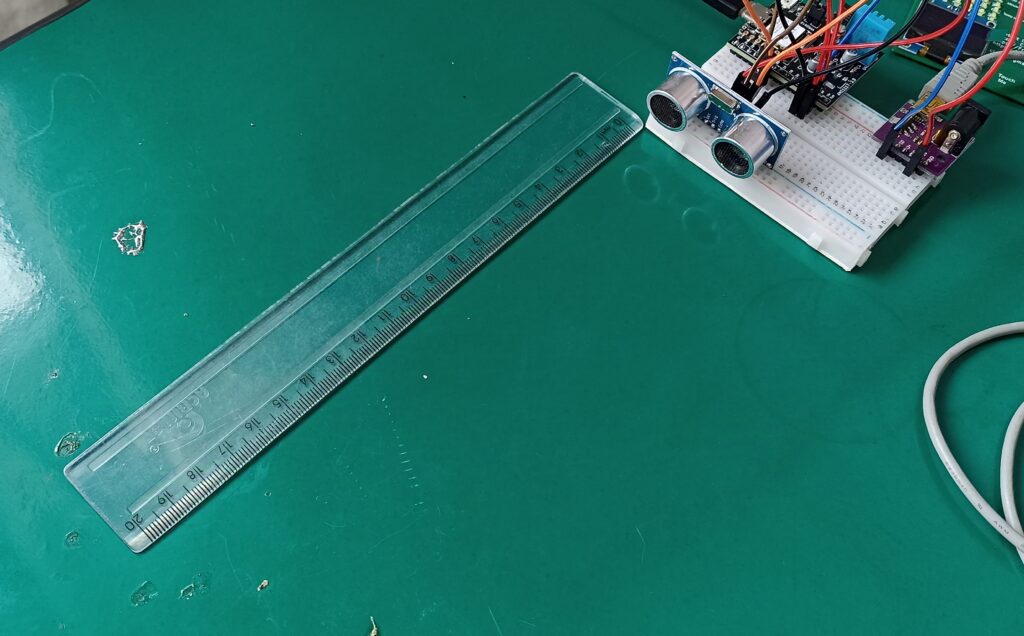
Without anything in front of the sensor the distance measured is around 1.58 meters, since there is a desk at the other side of the room I am at. Placing my hand in front of the sensor at 20cm gives me a reading of around 21-23cm, as seen below.
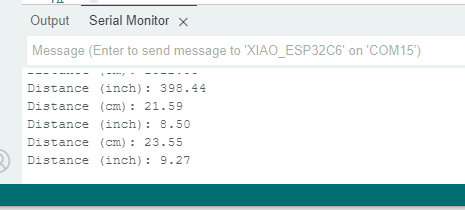
With my hand at 10cm it shows around 12.7cm, and so on. It is really not supposed to be very precise, it’s a time-of-flight measurement after all.
Final words
HC-SR04 is a neat little sensor used to measure distances, using the ultrasonic effect. You can buy one or more from my affiliate link, here. See you next time.
Leave a Reply