We are going to get to know the DS18b20 temperature sensor with ESP32-S2 Franzininho WiFi. The same code and connections can be applied to any Arduino-based board.
This sensor is manufactured by Analog devices and is capable of communicating with a microcontroller via a single wire, using the one-wire protocol. One-wire is a Serial protocol similar to i2c, but with lower speed and greater range.
This sensor is an old acquaintance of us makers/makers/students/curious , it comes in the format shown below. Three connections are required: +V (usually 3.3V), GND and signal.
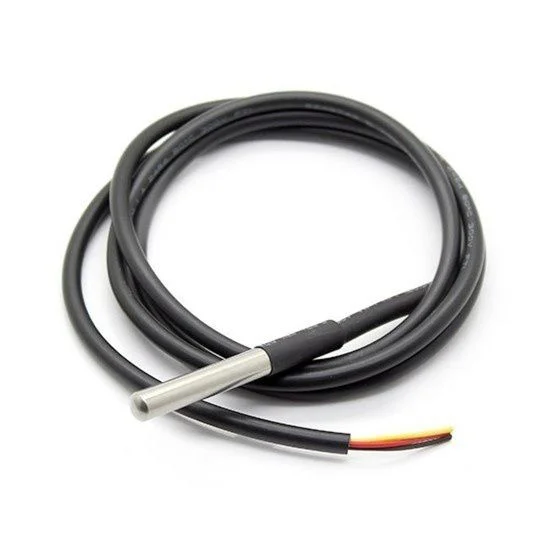
To prepare this article, I based myself on information from Robocore and CurtoCircuito. It is not necessary to install any special library, just have the OneWire library in your Arduino IDE. This code from Paul Stoffregen is all we need (see below).
OneWire ds(9); // on pin 9 (a 4.7K resistor is necessary)
void setup(void) {
Serial.begin(115200);
}
void loop(void) {
byte i;
byte present = 0;
byte type_s;
byte data[9];
byte addr[8];
float celsius, fahrenheit;
if ( !ds.search(addr)) {
Serial.println("No more addresses.");
Serial.println();
ds.reset_search();
delay(250);
return;
}
Serial.print("ROM =");
for( i = 0; i < 8; i++) {
Serial.write(' ');
Serial.print(addr[i], HEX);
}
if (OneWire::crc8(addr, 7) != addr[7]) {
Serial.println("CRC is not valid!");
return;
}
Serial.println();
// the first ROM byte indicates which chip
switch (addr[0]) {
case 0x10:
Serial.println(" Chip = DS18S20"); // or old DS1820
type_s = 1;
break;
case 0x28:
Serial.println(" Chip = DS18B20");
type_s = 0;
break;
case 0x22:
Serial.println(" Chip = DS1822");
type_s = 0;
break;
default:
Serial.println("Device is not a DS18x20 family device.");
return;
}
ds.reset();
ds.select(addr);
ds.write(0x44, 1); // start conversion, with parasite power on at the end
delay(1000); // maybe 750ms is enough, maybe not
// we might do a ds.depower() here, but the reset will take care of it.
present = ds.reset();
ds.select(addr);
ds.write(0xBE); // Read Scratchpad
Serial.print(" Data = ");
Serial.print(present, HEX);
Serial.print(" ");
for ( i = 0; i < 9; i++) { // we need 9 bytes
data[i] = ds.read();
Serial.print(data[i], HEX);
Serial.print(" ");
}
Serial.print(" CRC=");
Serial.print(OneWire::crc8(data, 8), HEX);
Serial.println();
// Convert the data to actual temperature
// because the result is a 16 bit signed integer, it should
// be stored to an "int16_t" type, which is always 16 bits
// even when compiled on a 32 bit processor.
int16_t raw = (data[1] << 8) | data[0];
if (type_s) {
raw = raw << 3; // 9 bit resolution default
if (data[7] == 0x10) {
// "count remain" gives full 12 bit resolution
raw = (raw & 0xFFF0) + 12 - data[6];
}
} else {
byte cfg = (data[4] & 0x60);
// at lower res, the low bits are undefined, so let's zero them
if (cfg == 0x00) raw = raw & ~7; // 9 bit resolution, 93.75 ms
else if (cfg == 0x20) raw = raw & ~3; // 10 bit res, 187.5 ms
else if (cfg == 0x40) raw = raw & ~1; // 11 bit res, 375 ms
//// default is 12 bit resolution, 750 ms conversion time
}
celsius = (float)raw / 16.0;
fahrenheit = celsius * 1.8 + 32.0;
Serial.print(" Temperature = ");
Serial.print(celsius);
Serial.print(" Celsius, ");
Serial.print(fahrenheit);
Serial.println(" Fahrenheit");
}
Schematic diagram and testing
Make the electrical connection as below, note that a 4k7 pull-up resistor is required between the signal and VCC. The sensor is powered by 3.3V.
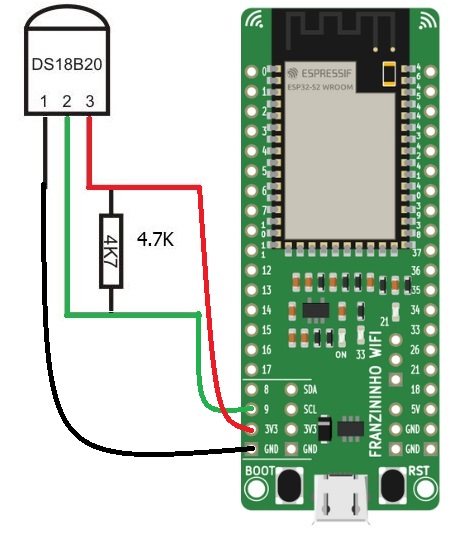
Downloading the code to the Franzininho WiFi board (with it connected via USB to the computer), we have the result below. Observe that the code let’s you know what type of chip is connected, in addition to the ROM content and current temperature.
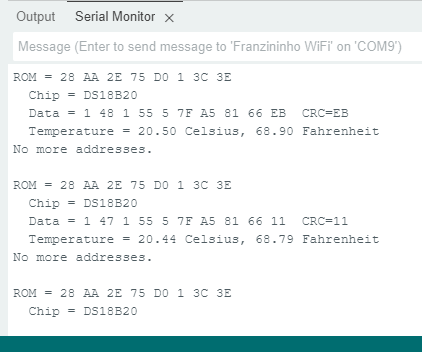
The DS18b20 is a simple but robust sensor and can even be applied in industrial environments (if correctly encapsulated). Its ease of use makes it ideal for small automations. Want to buy one? use my link here.
Do you want to know another temperature sensor? check out about SHT21.
Leave a Reply